As a web developer, you have likely come across the need to customize login pages in WordPress. Perhaps you have even considered adding reCAPTCHA to your login page to prevent spam and bots from using your website.
While there are several plugins available to accomplish this task, many developers prefer to avoid plugins, when possible, to maintain website performance and security.
In this article, we will explore how to create a custom login page with reCAPTCHA in WordPress without using a plugin.
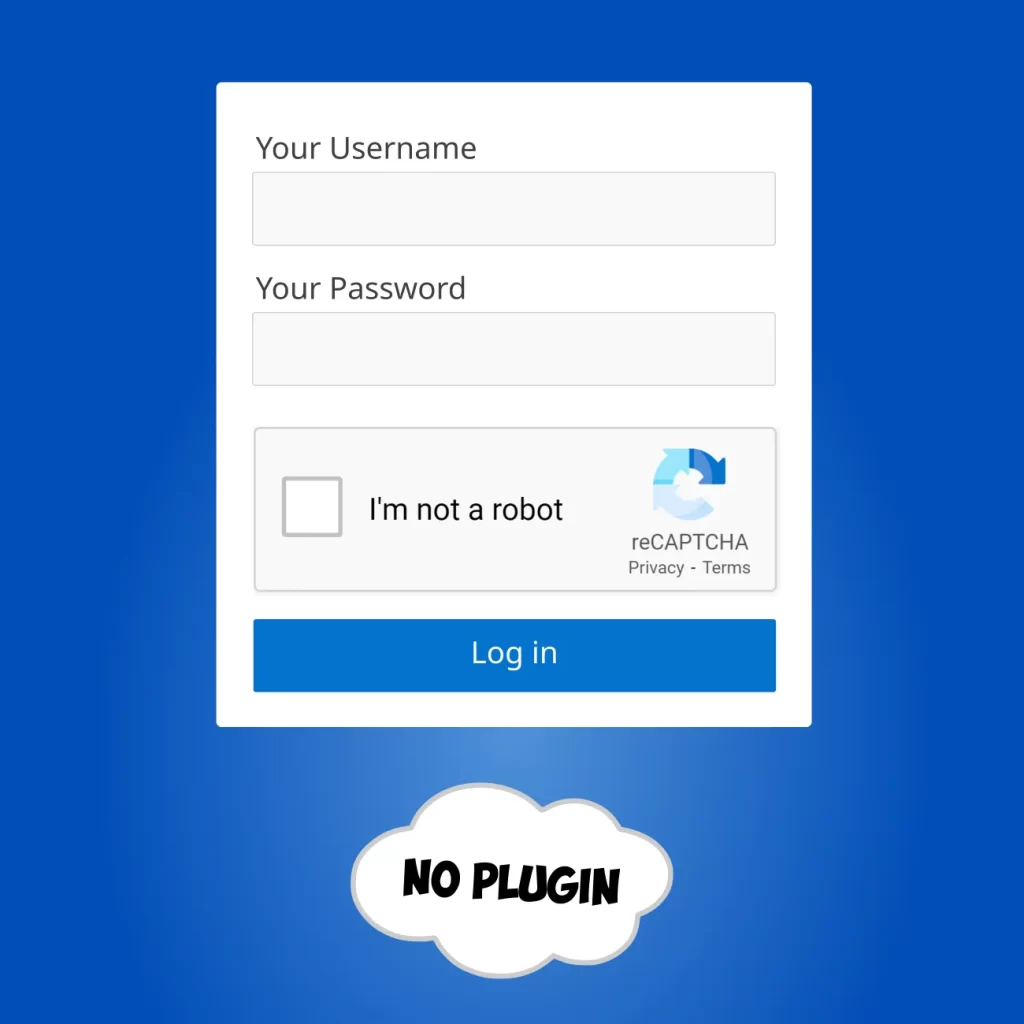
Custom login page with reCAPTCHA in WordPress without plugin
No coding skills are required to accomplish this task. Follow these simple steps:
- Register your website on the reCAPTCHA admin console and obtain the keys provided.
- Insert the received keys into code.
- Install the Code Snippets plugin to add the code to your website.
- Create a login page.
- Add the login shortcode to your page.
With these steps, you can easily add a reCAPTCHA login form to your website without needing any coding knowledge.
Step 1: Register your website on the reCAPTCHA admin console
Go to the reCAPTCHA admin console and create a new site. You will need to enter your website’s domain name and select the reCAPTCHA version you want to use.
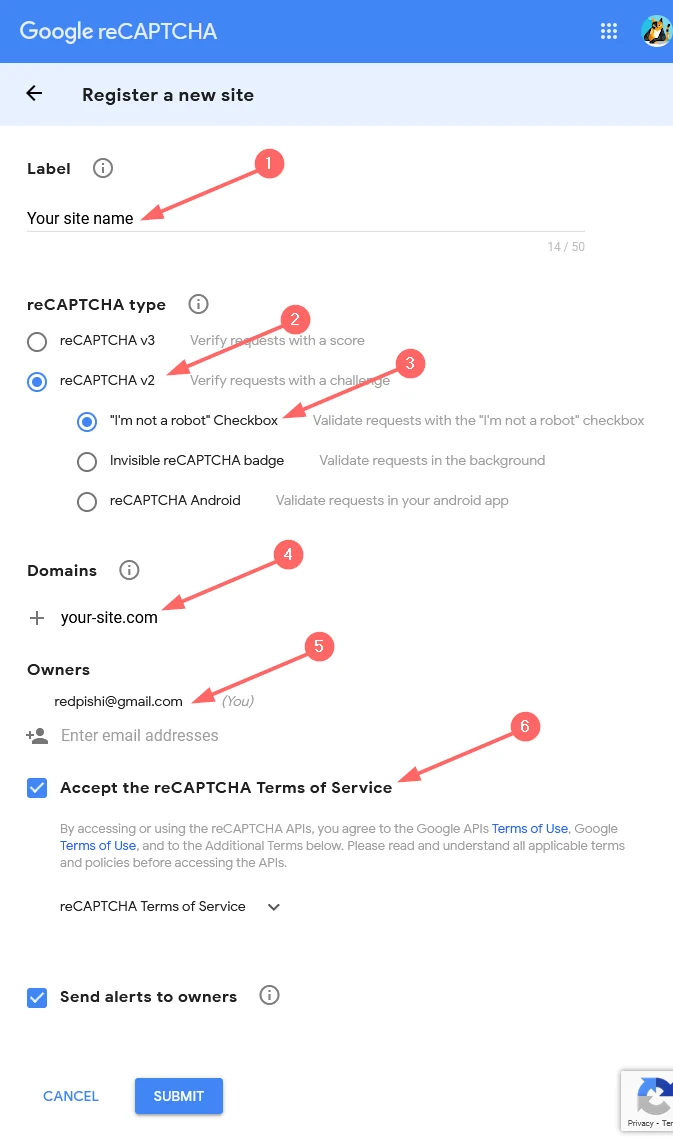
Fill out the above form as follows:
- Write a label for your site
- Choose reCAPTCHA v2
- Choose [“I’m not a robot” Checkbox]
- Enter your domain address.
- Enter the email address of the site administrator.
- Accept the reCAPTCHA Terms of Service
Then click on the submit button.
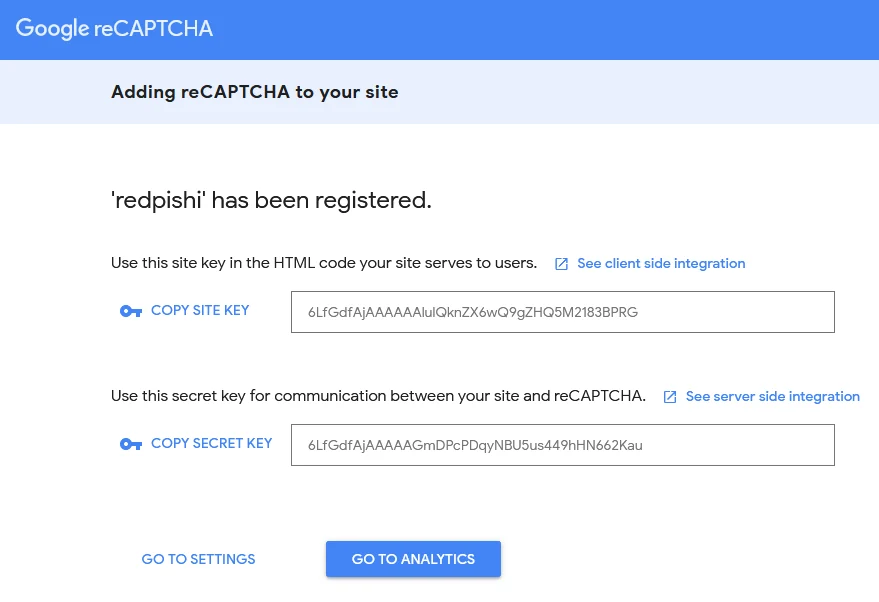
Step 2: Add the login shortcode code to your website
Write the site key and secret key in the code below and in the WordPress dashboard, go to Appearance ➡ Theme File Editor and insert it into the theme’s functions.php file and save it.
You must create a child theme before making any changes to functions.php
file. Otherwise, the applied changes will be lost after each update.
Create child theme in WordPress step by step [without plugin]
As an alternative method, you can use the Code Snippets plugin to insert your codes into WordPress.
/**
* Login shortcode with reCAPTCHA in WordPress by WPCookie
* https://redpishi.com/wordpress-tutorials/login-shortcode-with-recaptcha/
*/
function my_recaptcha_key(){
$sitekey= "000000000000000000000000000";
$secretkey= "00000000000000000000000000000000000";
return explode(",", $sitekey.",".$secretkey );
}
function add_recaptcha_on_login_page() {
echo '<div class="g-recaptcha brochure__form__captcha" data-sitekey="'.my_recaptcha_key()[0].'"></div>';
}
add_action('login_form','add_recaptcha_on_login_page');
function login_style() {
wp_register_script('login-recaptcha', 'https://www.google.com/recaptcha/api.js', false, NULL);
wp_enqueue_script('login-recaptcha');
echo "<style>p.submit, p.forgetmenot {margin-top: 10px!important;}.login form{width: 303px;} div#login_error {width: 322px;}</style>";
}
add_action('login_enqueue_scripts', 'login_style');
function captcha_login_check($user, $password) {
if (!empty($_POST['g-recaptcha-response'])) {
$secret = my_recaptcha_key()[1];
$ip = $_SERVER['REMOTE_ADDR'];
$captcha = $_POST['g-recaptcha-response'];
$rsp = file_get_contents('https://www.google.com/recaptcha/api/siteverify?secret=' . $secret . '&response=' . $captcha .'&remoteip='. $ip);
$valid = json_decode($rsp, true);
if ($valid["success"] == true) {
return $user;
} else {
return new WP_Error('Captcha-Invalid', __('<center>Captcha Invalid! Please check the captcha!</center>'));
}
} else {
return new WP_Error('Captcha-Invalid', __('<center>Captcha Invalid! Please check the captcha!</center>'));
}
}
add_action('wp_authenticate_user', 'captcha_login_check', 10, 2);
add_shortcode( 'login-form', 'loginform_func' );
function loginform_func( $atts ) {
$atts = shortcode_atts( array(
'logo' => '1',
), $atts, 'login-form' );
if ( is_user_logged_in() ) {
return "<p class='wpcookie-logged-user'>You are already logged in.</p>";
}
$content = wp_login_form(
array(
'echo' => false ,
'redirect' => get_home_url() ,
'label_username' => __( 'Your Username ' ),
'label_password' => __( 'Your Password' ),
'label_remember' => __( 'Remember Me' )
)
)."<a href=".esc_url( wp_lostpassword_url() )." style='font-size: 1rem;width: 100%;margin-left: 25px;'>Lost your password?</a>";
wp_register_script('login-recaptcha', 'https://www.google.com/recaptcha/api.js', false, NULL);
wp_enqueue_script('login-recaptcha');
$style1 = "<style>p.submit, p.forgetmenot {margin-top: 10px!important;}.login form{width: 303px;} div#login_error {width: 322px;}</style>";
$content = str_replace('<input type="submit" ','<div class="g-recaptcha brochure__form__captcha" data-sitekey="'.my_recaptcha_key()[0].'"></div><input type="submit" ',$content);
$error = "";
if (isset($_GET['reason'])) {
$er = "";
if($_GET['reason'] == 'Captcha-Invalid') {
$er = "Captcha Invalid! Please check the captcha!";
} else if ($_GET['reason'] == 'invalid_username' || $_GET['reason'] == 'incorrect_password' || $_GET['reason'] == 'empty_username' || $_GET['reason'] == 'empty_password') {
$er = "Invalid username or password, try again";
}
$error = '<p class="wpcookie_er">'.$er.'</p>';
}
$style2 = '
<style>
.wpcookie-login-form label {
display: block;
font-size: 1rem;
color: dimgrey;
}
.wpcookie-login-form input[type="text"], .wpcookie-login-form input[type="password"] {
height: 2rem;
width: 303px;
border: 1px solid #e7e7e7;
border-radius: 5px;
background-color: #ffffff26;
}
.wpcookie-login-form p.wpcookie_er {
font-size: 1rem;
color: #b11414;
}
.wpcookie-login-form input[type="submit"] {
color: white;
background-color: #4e8ef5;
outline: none;
border: navajowhite;
padding: 0.7rem;
width: 303px;
margin-top: 15px;
border-radius: 5px;
transition: all 0.5s ease;
cursor: pointer;
}
.wpcookie-login-form input[type="submit"]:hover {
background-color: #1d3eab;
}
.wpcookie-login-form {
width: 350px;
margin: auto;
background-color: #ffffff;
border-radius: 5px;
padding: 20px;
display: flex;
justify-content: center;
flex-direction: column;
flex-wrap: nowrap;
align-items: center;
}
p.wpcookie-logged-user {
width: fit-content;
font-weight: bold;
border-radius: 5px;
padding: 15px;
background-color: #ffffffc2;
color: black!important;
margin: auto;
}
</style>
';
$logo_url = esc_url( wp_get_attachment_image_src( get_theme_mod( 'custom_logo' ), 'full' )[0]) ;
$logo ='<img src="'.$logo_url.'" alt="logo" style="padding: 15px; height: 106px;">';
if ($atts["logo"] == 0 ) { $logo = ""; }
return "{$style2}{$style1}<div class='wpcookie-login-form'>{$logo}{$error}{$content}</div>";
}
add_filter('login_redirect', 'my_login_redirect', 10, 3);
function my_login_redirect($redirect_to, $requested_redirect_to, $user) {
if (is_wp_error($user)) {
$error_types = array_keys($user->errors);
$error_type = 'both_empty';
if (is_array($error_types) && !empty($error_types)) {
$error_type = $error_types[0];
}
if(isset($_SERVER['HTTP_REFERER']) ) {
$location = strtok($_SERVER['HTTP_REFERER'], '?');
if ( str_contains( $location, "wp-login.php") ) {return;}
wp_redirect( $location . "?login=failed&reason=" . $error_type );
exit;
} else return;
} else {
return home_url();
}
}
By inserting the above code into WordPress, reCAPTCHA will be added to the default WordPress login form, and the [login-form] shortcode will be registered on your site.
If you are already using the code in the article Add reCAPTCHA to WordPress without plugins, you must delete the common part of these two codes from the above code, otherwise the above code will not be activated on your site.
This shortcode has only one argument, if you want to remove the site logo from the form, set the logo to 0.
[login-form logo="0"]
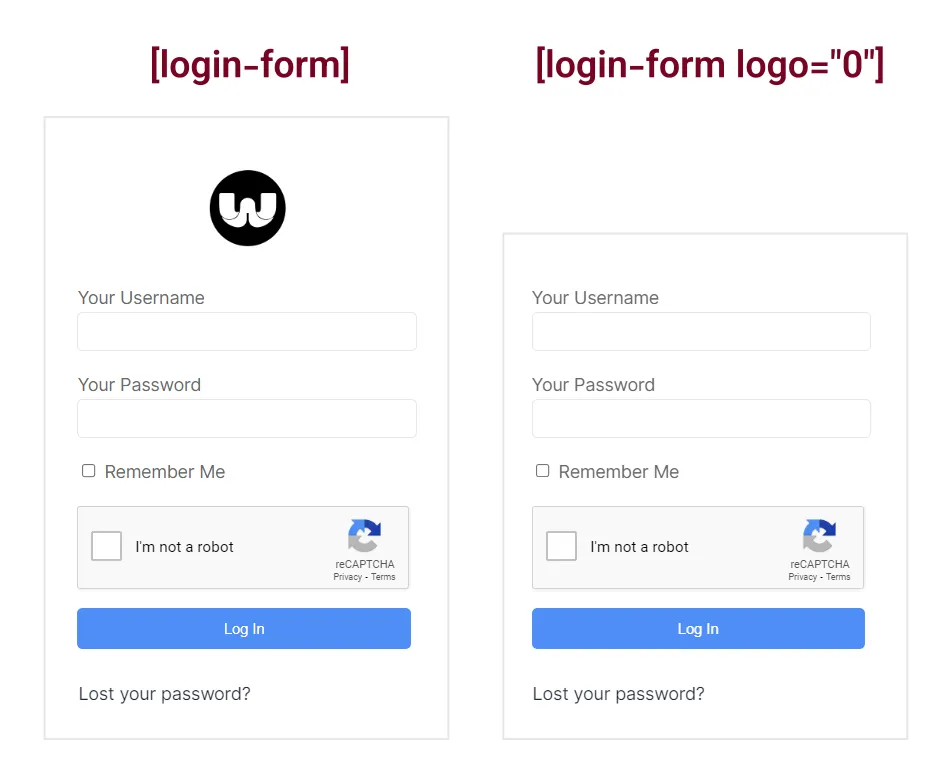
Step 3: Design your login page and add the shortcode to it
Create a new page and design it according to your taste and finally add the above shortcode to it.
If you use the Gutenberg page builder, you can add a cover block to the page, upload your desired photo and finally add the [login-form] shortcode to it.
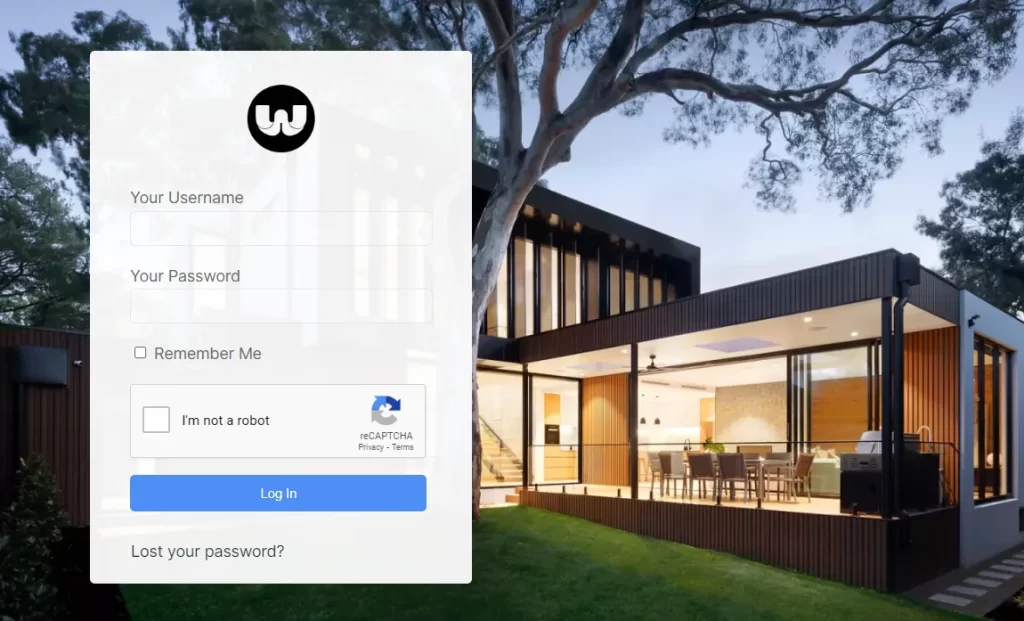
Congratulations, you have successfully created a custom login page with reCAPTCHA in WordPress without using a plugin! By customizing your login page and adding reCAPTCHA, you have improved your website’s security and prevented spam and bots from accessing your content.
If this article is difficult for you to read in text, you can watch the video version below.
Hi!
Is it possible somehow to get wordpress to automatically redirect to the new login page you have created when a visitor goes to /wp-admin directory?
Hi Jonas
I have already covered this topic in a previous post. You can find the instructions on how to redirect the WordPress login page in this link:
[How to change the WordPress admin URL without plugins].
Look for the section titled Redirecting the WordPress login page and follow the steps there.
Thank you.
Question: This doesn\’t work with the password reset link. Can you add that, please? Also, when someone enters their username to reset their password (when the password reset email is sent), they are routed back to the login page. Is there a way you can add a notice that says \”Password reset requested. Please check your email\” or something similar?
Finally, I was having issues with redirect_to from get (the webpage wasn\’t picking it up) so I changed the following in the wp_login_form array:
\’redirect\’ => ( is_ssl() ? \’https://\’ : \’http://\’ ) . $_SERVER[\’HTTP_HOST\’] . $_SERVER[\’REQUEST_URI\’] ,
Now it works!
Hi Maya,
I love your tutorials & guides. Thank you so much for this precious content. Is it also possible to add a quiz to the login form—instead of a reCAPTCHA?
Something like:
Please enter the solution to this calculation:
15+5=?
This would also block bot logins and reduce load time and connections. How would a solution for the functions.php look like?
Best,
Jakob
Hey Jakob! Absolutely, you can totally opt for a mathematical captcha instead of Google\’s reCAPTCHA. However, it\’s important to note that the security level might be a tad lower compared to Google\’s reCAPTCHA. If I receive more requests for this type of captcha, I\’ll be more than happy to whip up a new article about it!
Thank you !